Video version:
FizzBuzz is the most used problem in developer jobs interviews to filter out people on
Context

When any job position is open, dozens if not hundreds of people apply for them. Especially if we talk about developer jobs, where they pay is big (so I heard…).
The normal way to deal with this is filtering people out in a fast and simple way, for example, by making them solve problems.
You may think that those problem may be extremely hard to solve, but in fact they are easy (at least on earlier stages). And one of the most used problem, if not the most used, it is the FizzBuzz challenge.
What’s the problem?
The FizzBuzz problem is pretty simple. With small variations, it ask you to print every number from 0 to 100, and when the number is divisible by 3 (3, 6, 9, 12…) you have to print “Fizz”. When it is divisible by 5 (5, 10, 15, 20..), print “Buzz.”
When both conditions apply (15, 30, 45…), print “FizzBuzz” instead.
Pretty easy, right?
Time to solve it
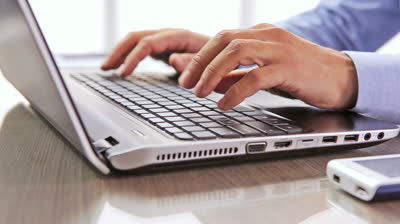
As I said, this would be your first exercise in most of your junior interviews, so why not try to solve it on your own?
Print every number from 0 to 100, writing Fizz then the number is divisible by 3, Buzz when it is divisible by 5, and FizzBuzz when it is divisible by 15.
Stop reading and do it on your own. Even if you don’t succeed, try it.
A few tips
Ok, you tried but you didn’t succeed. I’m
Don’t keep reading if you didn’t try first!
Tip 1: You need to print numbers from 0 to 100. While you can use many ways to iterate over a bunch of numbers, you need to think the most and easier way for this part.
Tip 2: The condition to print Fizz, Buzz or FizzBuzz is that the current number is divisible by 3, 5 or 3 and 5. That is, you can divide them and doesn’t have any decimals as remainder.
Tip 3: Mind the order of your operations!
My solution
# Exercise: Fizzbuzz. From 0 to 100, print all numbers. If number is divisible by 3, print 'Fizz', if number is divisible by 5, print 'Buzz', if divisible by 3 and 5, print 'FizzBuzz'. Else, print number for number in range(0, 101): if (number % 3 == 0) and (number % 5 == 0): print(f'{number}: FizzBuzz') elif (number % 3 == 0): print(f'{number}: Fizz') elif (number % 5 == 0): print(f'{number}: Buzz') else: print(number)
First, we iterate from 0 to 100. Yes, the for loop is “for number in range(0, 101)”, having 0 as start number and 101 as stop, but not including 101.
Then, we check the number remainder with modulus.
Caution! The comparison of 3 AND 5 conditions we see at line 4 has to be the first one. If we put it that comparison at the end, the code will never reach it, ending that iteration when the number is divisible by 3 OR 5 (move around the code if you want to check it).
Then, if no conditions are True (is not divisible by 3 or 5), just print the number.
So… did you solved the problem? Congratulations!
This is the first of many exercises I’m gonna propose, explain and solve. Compare your solution with mine. Maybe I solve it in a more simple way. Maybe you solved it with less code than I.
If you didn’t managed to solve it… Congratulations!
Yes, congratulations. You tried to solve it and, despite not managing to solve it on your own, now you know how to solve it, and why we use this way to solve. You have learnt how for…range loops work, how to compare numbers and more.
Now come back in one or two hours, or the next day, and try to solve it without looking at the solution. When you can do it, go for the next exercise!
The REPL with my code: https://repl.it/@DavidMM1707/06-Fizzbuzz
Find me on Twitter at: https://twitter.com/DavidMM1707
My GitHub: https://github.com/david1707
Learn more about FizzBuzz and why is still important:
https://hackernoon.com/why-im-still-using-fizz-buzz-to-hire-software-developers-7e31a89a4bbf
Seventh lesson: 07 – Guess the number